How Builders Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
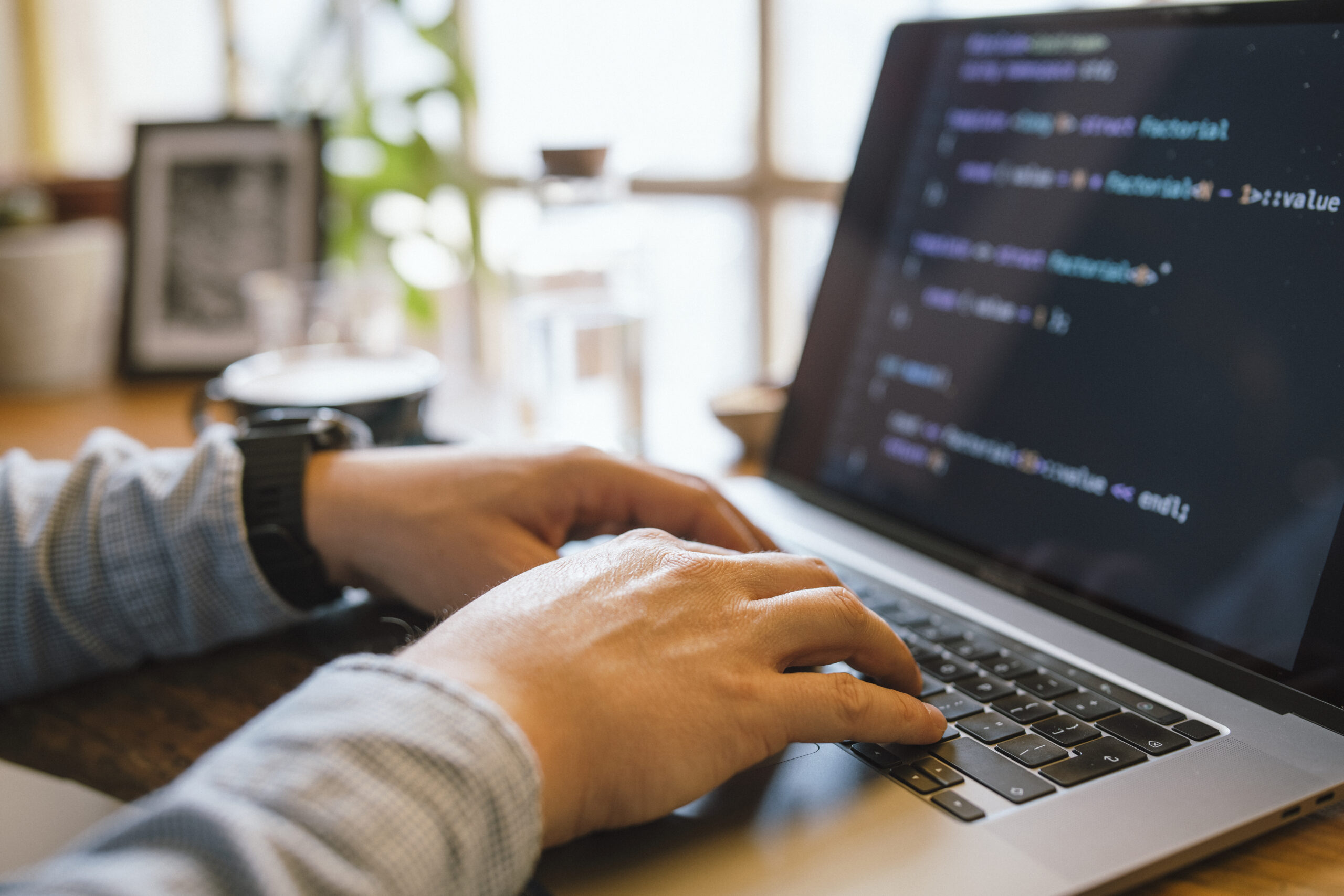
Debugging is Just about the most vital — but generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about being familiar with how and why things go wrong, and Studying to Believe methodically to solve difficulties proficiently. No matter whether you're a novice or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productiveness. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, understanding how to connect with it properly in the course of execution is Similarly significant. Modern day advancement environments come Geared up with effective debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Just take, one example is, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code to the fly. When employed the right way, they Allow you to notice specifically how your code behaves all through execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Handle programs like Git to be familiar with code history, discover the exact second bugs have been released, and isolate problematic variations.
Ultimately, mastering your tools indicates going over and above default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time you may commit fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — techniques in productive debugging is reproducing the challenge. Ahead of jumping into the code or making guesses, builders will need to make a steady atmosphere or state of affairs wherever the bug reliably seems. With out reproducibility, correcting a bug will become a match of likelihood, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more detail you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating comparable person interactions, or mimicking method states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions included. These tests not simply help expose the trouble and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging instruments extra correctly, exam potential fixes safely, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when a thing goes Erroneous. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, particularly when under time force, glance at the main line and promptly commence making assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into search engines like yahoo — read and recognize them initial.
Split the error down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology on the programming language or framework you’re using. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in People conditions, it’s essential to look at the context by which the error transpired. Test associated log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These typically precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint challenges more quickly, lower debugging time, and turn into a extra effective and assured developer.
Use Logging Properly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When employed properly, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on under the hood without needing to pause execution or stage with the code line by line.
A great logging method begins with realizing what to log and at what level. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for in-depth diagnostic facts during development, Facts for typical situations (like effective start-ups), Alert for likely troubles that don’t split the appliance, Mistake for genuine challenges, and Lethal when the technique can’t continue on.
Steer clear of flooding your logs with abnormal or irrelevant information. A lot of logging can obscure essential messages and decelerate your technique. Give attention to important situations, condition adjustments, input/output values, and important determination points as part of your code.
Format your log messages Plainly and constantly. Incorporate context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in generation environments exactly where stepping as a result of code isn’t achievable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Ultimately, clever logging is about equilibrium and clarity. That has a properly-considered-out logging tactic, you may reduce the time it takes to identify difficulties, achieve deeper visibility into your applications, and Enhance the overall maintainability and trustworthiness of one's code.
Imagine Just like a Detective
Debugging is not merely a technical activity—it is a type of investigation. To proficiently identify and resolve bugs, builders will have to approach the process just like a detective fixing a thriller. This mindset assists break down advanced issues into manageable areas and abide by clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, collect just as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be producing this actions? Have any improvements not long ago been manufactured on the codebase? Has this concern occurred right before less than related conditions? The objective would be to slender down options and discover possible culprits.
Then, test your theories systematically. Endeavor to recreate the challenge within a managed natural environment. Should you suspect a particular purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the reality.
Spend shut interest to compact information. Bugs frequently disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might cover the real difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Producing checks is among the most effective approaches to increase your debugging competencies and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that offers you self-confidence when creating adjustments to the codebase. A very well-analyzed software is easier to debug because it permits you to pinpoint specifically the place and when a difficulty happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you promptly know the place to search, substantially lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a attribute properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the annoying guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, faster and even more reliably.
Acquire Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A brief stroll, a coffee break, or simply switching to another endeavor for ten–quarter-hour can refresh your emphasis. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Vitality along with a clearer mentality. You would possibly out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below limited deadlines, however it essentially results in speedier and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can educate you a thing important in the event you take read more some time to mirror and assess what went Completely wrong.
Start by asking oneself a number of essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving forward.
Documenting bugs can be a fantastic routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you acquired. After some time, you’ll begin to see designs—recurring problems or common issues—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly master from their errors.
Eventually, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and able developer. The following time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at Anything you do.